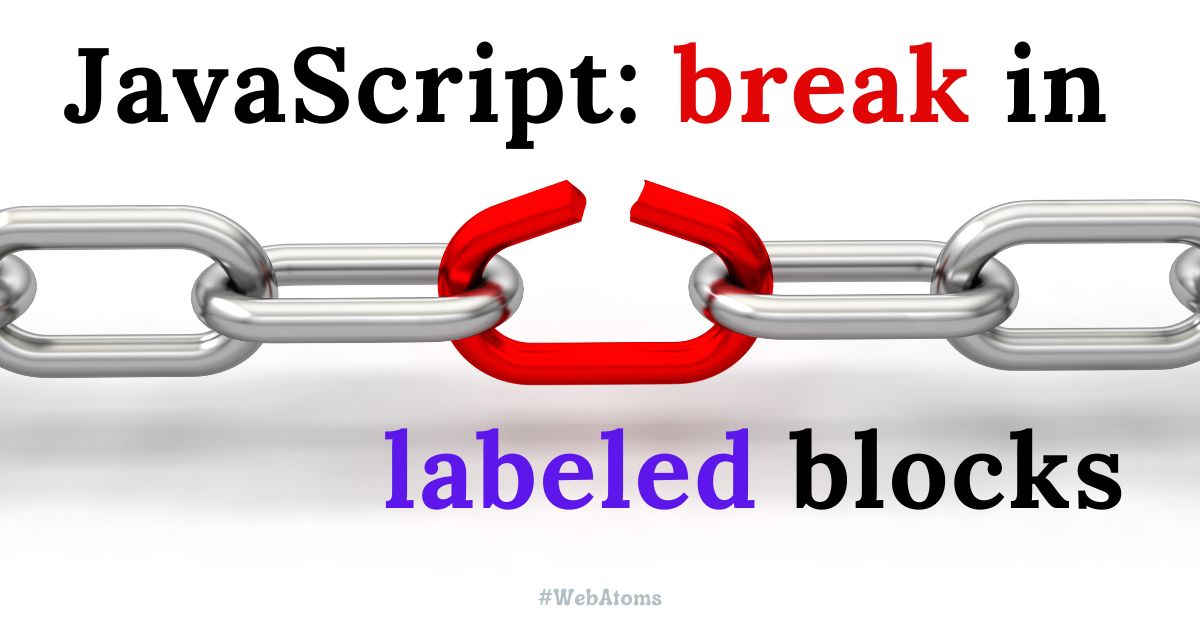
'BREAK' Statement in 'labeled' blocks.
We have used break
statement in while
loop, for
loop and switch
statements. But we can also use break
statement within a labeled block. Let's take a look at the example:-
What would be the output of the below code snippet?
{
var a = 1;
named: {
a++;
break named;
a++;
}
console.log(a);
}
Output:
2
A break
statement should be nested in the referenced label. Here 'named' is the label and break <label-name>
is the syntax used to break or bypass the logic in the labeled block named
.
Can we use it like the GOTO
statement in the labeled blocks? No. Break
and Continue
can be used in the loops to give you the civilized form of GOTO
statement. Let's look at the example
What would be the output of the below code snippet?
label1: {
console.log("I am in Label 1");
break label2:
}
label2: {
console.log("I am Label 2");
}
Output:
SyntaxError: Undefined label 'label2'
So, as we can see in the above example, we cannot jump or goto label2
from label1
using break
.
Can I use continue
in the labeled
blocks? NO. Let's us evaluate the same example and replace break
with continue
.
{
var a = 1;
named: {
a++;
continue named; //break replaced with `continue`
a++;
}
console.log(a);
}
Output:
SyntaxError: Illegal continue statement: 'named' does not denote an iteration statement
Can we have nested
labeled blocks? Yes, we can.
firstLabel: {
secondLabel: {
console.log("Hi WebAtoms");
break firstLabel;
console.log("Will you print me?");
}
console.log("Am I in scope?");
}
Output:
Hi WebAtoms
If you look at the snippet, it breaks out of both firstLabel
and secondLabel
. If I replace the break
statement as shown below:-
firstLabel: {
secondLabel: {
console.log("Hi WebAtoms");
break secondLabel;
console.log("Will you print me?");
}
console.log("Am I in scope?");
}
Output:
Hi WebAtoms
Am I in scope?
Can I use break
within functions nested in a loop or labeled block?
NO, If we try to use break
statement within functions that are nested in a loop or labeled block, it will generate SyntaxError
. Which also implies, labeled blocks
cannot contain functions
.
Hope you have learnt something new today. Happy learning.
![]() | ![]() | ![]() | ![]() |
Like | Comment | Save | Share |