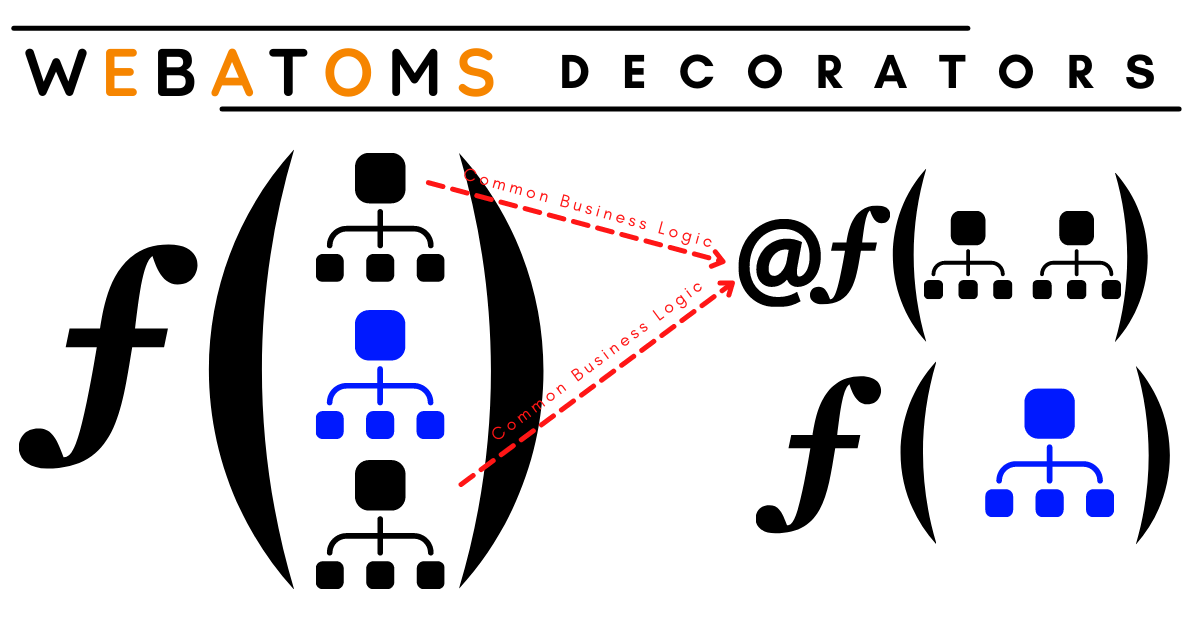
What are Function/Method Decorators?
Function decorators allow us to add extra feature to your existing function i.e. it allows to change behavior of your existing function at compile time.
WebAtoms: Load Decorator
Load decorator makes loading of async data very easy. Let's assume that we want to load countries and then selected state. It reports exceptions and cancels previous operation.
export default class SignupViewModel extends AtomViewModel {
/** This gets called on initialization */
@Load({ init: true })
public async loadCountries() {
this.countries =
await this.restService.getCountries();
this.selectedCountry = this.countries
.find((x) => x.value === "IN").value;
}
/** This gets called when `this.selectedCountry` changes */
@Load({ watch: true /* watch */ })
public async loadStates(ct: CancelToken) {
const country = this.selectedCountry;
// pass cancel token
// to automatically cancel previous
// incomplete request
this.states =
await this.restService.getStates(country, ct);
}
}
If you look in the above logic, we have a decorator @Load({init: true})
, which will be executed during the initialization (only once
) of view model (we can use init
on multiple methods) and another @Load({watch:true})
, which will watch for changes in the country selected and fire the next operation i.e. getting the states
based on country
selected.
What is Debouncing?
It is a programming technique to limit the number of times a long-drawn-out function gets called, which stalls the performance of your web page.
How can we achieve Debouncing using WebAtoms?
Let's look at the example below.
export default class SignupViewModel extends AtomViewModel {
@Load({
/** watch for changes */
watch: true,
/** wait as user might still be typing */
watchDelayInMS: 500
})
public async loadCities(ct: CancelToken) {
const search = this.search;
/** cancellation of previous request is tracked here */
this.cities = await
this.restService.searchCities(search, ct);
}
}
As we look in the above logic, we are watching for any changes (user input) to the City
. We have introduced a delay of 500ms as user might still be typing, ct
is a cancel token which is tracked and only the last call result is returned i.e. if the debounced method/function is called only the latest call will result in the method call, the other ones will be discarded thereby limiting the number of times the method is invoked and improving the performance.
![]() | ![]() | ![]() | ![]() |
Like | Comment | Save | Share |