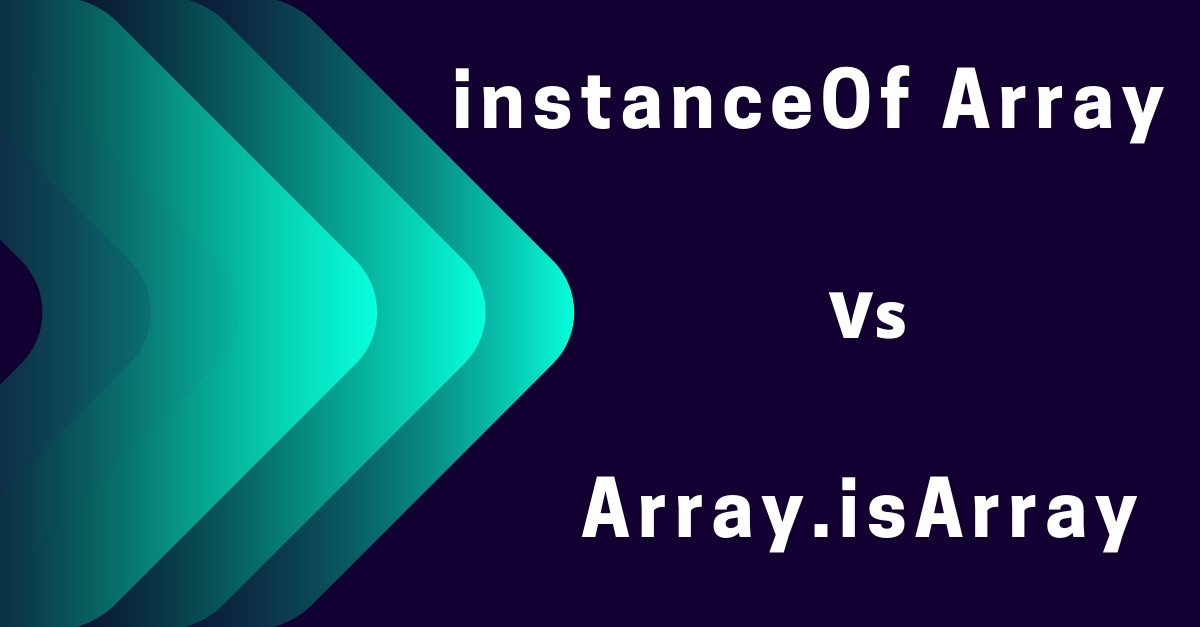
There are two major differences between instanceOf Array
and Array.isArray
Cross Context Object
In browser, when you access object from different window (such as different iframe from same domain), instanceOf Array
will return false. However Array.isArray
will return true.
For example,
var iframe = document.createElement('iframe');
document.body.appendChild(iframe);
iArray = window.frames[window.frames.length-1].Array;
var arr = new iArray(1,2,3); // [1,2,3]
// Correctly checking for Array
Array.isArray(arr); // true
// Considered harmful, because doesn't work through iframes
arr instanceof Array; // false
Why?
Well internally, all root level (global) functions/constructors such as Array
, Object
are isolated under the currently executing contexts. Two browser windows or two iframes will have different context and object created in one context will have prototype associated with the context.
When object is accessed in different context, instanceOf
will fail to identify object's prototype chain in current context.
Created with Object.create
var a = Object.create( Array.prototype, {});
a.push(1);
a.push(2);
var b = [];
b.push(1);
b.push(2);
console.log(a.join()); // it will display '1,2'.
console.log(b.join()); // it will display '1,2'.
Array.isArray(a); // false
Array.isArray(b); // true
a instanceOf Array; // true
b instanceOf Array; // true
Why?
With Object.create
, Array.prototype is present in prototype chain of a
, so instanceOf Array
will be true, as in the context of JavaScript, a has prototype of Array. So all the methods of Array
will work correctly on a
as those methods are generic.
But Array.isArray
does not check for prototype, it checks if it was created using Array
constructor, which internally is a separate type of object in the language it was implemented. Such as in V8, Array
constructor will create an object of class V8Array
which inherits V8Object
. And Object.create
will create V8Object
. Array.isArray
method in V8 will check if the receiver is instance of V8Array
class in C++. Similarly every JavaScript engine will check natively, what kind of object it is, rather then checking prototype.
![]() | ![]() | ![]() | ![]() |
Like | Comment | Save | Share |